Join our newsletter
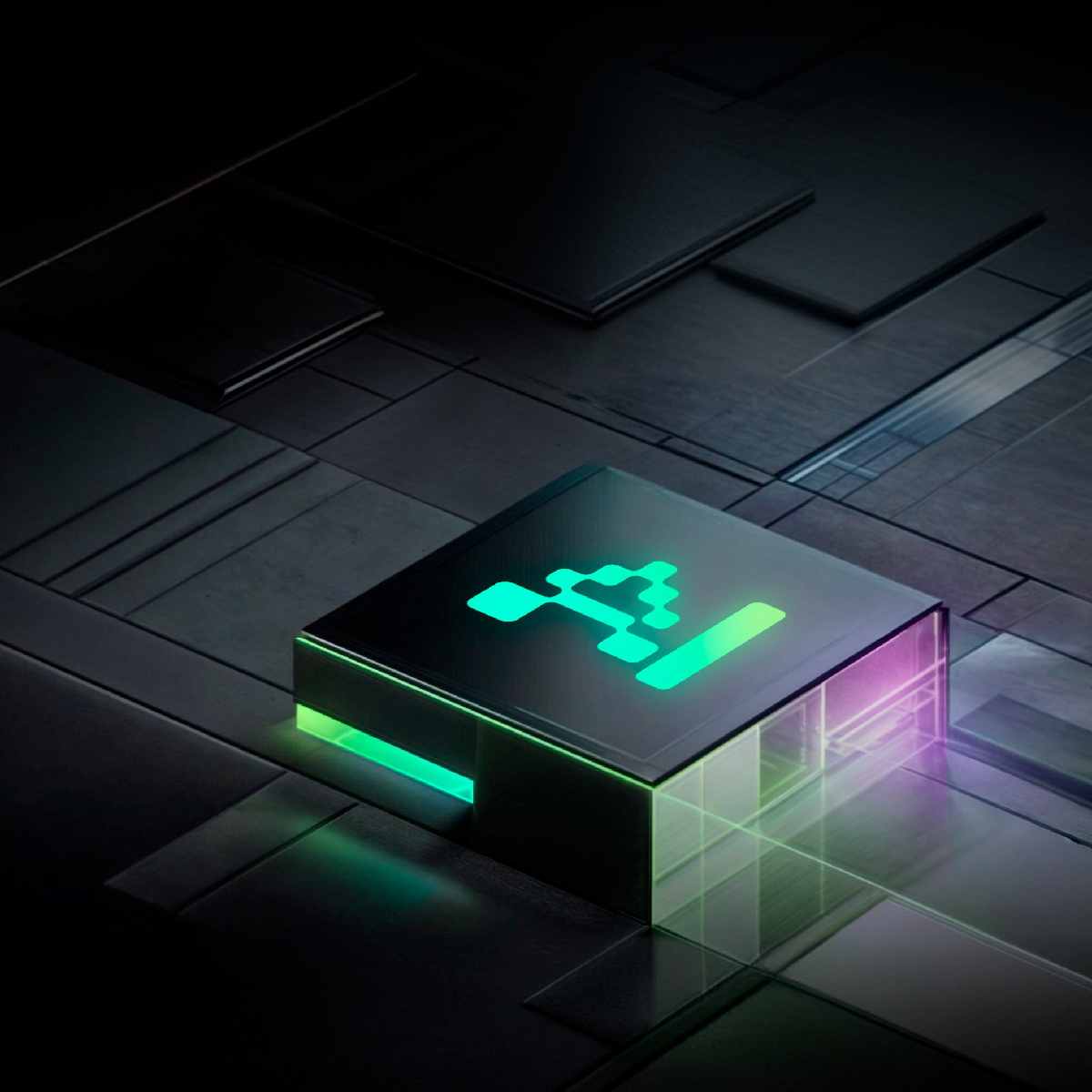
Transformers is a powerful tool for sentiment analysis, a natural language processing task aimed at determining the sentiment expressed within a piece of text.
Here's a breakdown of how Transformers excel in sentiment analysis:
By leveraging these models and the capabilities of the Transformers library, developers can build robust and effective sentiment analysis systems for various applications, such as social media monitoring, customer feedback analysis, and market research.
import pandas as pd
import numpy as np
import torch
From transformers import AutoTokenizer, AutoModelForSequenceClassification, Trainer, TrainingArguments
Load the dataset:
From datasets import load_dataset
dataset = load_dataset("imdb")
print(dataset)
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
def tokenize_function(examples):
return tokenizer(examples["text"], padding="max_length", truncation=True)
tokenized_datasets = dataset.map(tokenize_function, batched=True)
From datasets import train_test_split
train_testvalid = tokenized_datasets['train'].train_test_split(test_size=0.2)
train_dataset = train_testvalid['train']
valid_dataset = train_testvalid['test']
Load the model:
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased", num_labels=2)
If the case study is positive and negative, we recommend leaving the categories in 2. Should you need more categories, add the number.
training_args = TrainingArguments( output_dir="./results", evaluation_strategy="epoch", save_strategy="epoch", num_train_epochs=3, per_device_train_batch_size=16, per_device_eval_batch_size=16, warmup_steps=500, weight_decay=0.01, )
**Create a Trainer instance:**
trainer = Trainer(
model=model,
args=training_args,
train_dataset=tokenized_train,
eval_dataset=tokenized_test,
)
trainer.train()
predictions = trainer.predict(valid_dataset)
print(predictions)
You can evaluate the model's performance using metrics like accuracy, precision, recall, and F1-score.
metrics = trainer.evaluate()
print(metrics)
By following these steps and considering the additional points, you can build effective sentiment analysis models using the Transformers library.